Converting HEX Color To SolidColorBrush In WinUI 3
As I was building OpenSpartan Workshop for Windows I needed the ability to convert a hexadecimal HTML color code to a SolidColorBrush
object. Unlike with previous UI frameworks, WinUI 3 (which is what I use) doesn’t have a built-in construct for this kind of conversion. Naturally, I had to improvise.
Let’s start by taking a peek at what the structure of a hexadecimal (HEX) color code is:
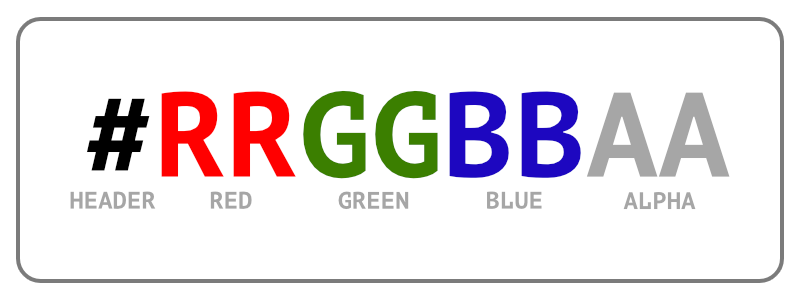
There are four core components to the code (other than the header):
- The red color identifier, that can be between 0 and 255.
- The green color identifier, also between 0 and 255.
- The blue color identifier, just as the previous ones - between 0 and 255.
- The alpha (transparency) identifier, with the same value ranges.
Every identifier is stored in a single byte (8 bits). Because each byte can be between 0 and 255, that means that for every color we get 256 variations, giving us a total of 16,777,216 possible colors. That is, if we don’t account for alpha channel, that can also be between 0 (00
) and 255 (FF
), where the former is fully transparent and the latter is fully opaque.
For us to be able to convert these individual values into a SolidColorBrush
, I need to split up the string into its component chunks and then use Color.FromArgb
to convert them into the destination object. Like this:
internal class ColorConverter
{
public static SolidColorBrush FromHex(string hex)
{
hex = hex.TrimStart('#');
byte a = 255; // Default alpha value
byte r = byte.Parse(hex.Substring(0, 2), System.Globalization.NumberStyles.HexNumber);
byte g = byte.Parse(hex.Substring(2, 2), System.Globalization.NumberStyles.HexNumber);
byte b = byte.Parse(hex.Substring(4, 2), System.Globalization.NumberStyles.HexNumber);
if (hex.Length == 8)
{
a = byte.Parse(hex.Substring(6, 2), System.Globalization.NumberStyles.HexNumber);
}
return new SolidColorBrush(Color.FromArgb(a, r, g, b));
}
}
Notice that I am using a default alpha channel value (255
) if there is no HEX value for it encoded in the actual code passed into FromHex
.
And just like that, I am able to use HEX color codes in my application without taking dependency on anything else.